Slackコミュニティに
無料で参加する
Flutterラボの
プレミアム会員になる
【Flutter】scroll_to_idパッケージの使い方
2020.12.16
HTMLのようにidを指定して、そこまでスクロールすることができるパッケージ『scroll_to_id』の使い方を紹介します。
pubspec.yamlに追記して、パッケージを取得します。
dependencies:
scroll_to_id: any
ScrollToIdのインスタンスを取得します。
final ScrollToId scrollToId = ScrollToId();
InteractiveScrollViewerウィジェットを用意し、childrenプロパティにScrollContentを入れていきます。
スクロールさせるときに、idプロパティに指定した値を使用します。
InteractiveScrollViewer(
scrollToId: scrollToId,
children: <ScrollContent>[
ScrollContent(
id: 'a',
child: Container(
color: Colors.red,
width: 300,
height: 200,
)
),
ScrollContent(
id: 'b',
child: Container(
color: Colors.green,
width: 300,
height: 200,
)
),
],
)
あとは、スクロールさせるだけです。
通常のanimateToとjumpToと使い方は同じで、offsetではなくidを指定してスクロール位置を決定することができます。
/// animate
scrollToId.animateTo(
'b',
duration: Duration(milliseconds: 500),
curve: Curves.ease
);
/// jump
scrollToId.jumpTo('b');
実行例コードを載せておくので、参考にしてみてください。
import 'package:flutter/material.dart';
import 'package:scroll_to_id/scroll_to_id.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
/// Create ScrollToId instance
final ScrollToId scrollToId = ScrollToId();
List<Color> _colorList = [
Colors.green,
Colors.red,
Colors.yellow,
Colors.blue
];
/// Generate 10 Container
/// Case [Axis.horizontal] set buildStackHorizontal() to body
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Scroll to id',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: const Text('Scroll to id'),
),
body: buildStackVertical(),
),
);
}
/// [Axis.vertical]
Widget buildStackVertical() {
return Stack(
alignment: Alignment.topRight,
children: [
InteractiveScrollViewer(
scrollToId: scrollToId,
children: List.generate(10, (index) {
return ScrollContent(
id: '$index',
child: Container(
alignment: Alignment.center,
width: double.infinity,
height: 200,
child: Text(
'$index',
style: TextStyle(color: Colors.white, fontSize: 50),
),
color: _colorList[index % _colorList.length],
),
);
}),
),
Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.white, width: 3),
),
child: Column(
mainAxisSize: MainAxisSize.min,
children: List.generate(10, (index) {
return GestureDetector(
child: Container(
width: 100,
alignment: Alignment.center,
height: 50,
child: Text(
'$index',
style: TextStyle(color: Colors.white),
),
color: _colorList[index % _colorList.length],
),
onTap: () {
/// scroll with animation
scrollToId.animateTo('$index',
duration: Duration(milliseconds: 500),
curve: Curves.ease);
/// scroll with jump
// scrollToId.jumpTo('$index');
},
);
}),
),
)
],
);
}
/// [Axis.horizontal]
Widget buildStackHorizontal() {
return Column(
children: [
Container(
width: double.infinity,
decoration: BoxDecoration(
border: Border.all(color: Colors.white, width: 3),
),
child: Row(
mainAxisSize: MainAxisSize.min,
children: List.generate(10, (index) {
return Expanded(
child: GestureDetector(
child: Container(
alignment: Alignment.center,
height: 80,
child: Text(
'$index',
style: TextStyle(color: Colors.white),
),
color: _colorList[index % _colorList.length],
),
onTap: () {
/// scroll with animation
scrollToId.animateTo('$index',
duration: Duration(milliseconds: 500),
curve: Curves.ease);
/// scroll with jump
// scrollToId.jumpTo('$index');
},
),
);
}),
),
),
Expanded(
child: InteractiveScrollViewer(
scrollToId: scrollToId,
scrollDirection: Axis.horizontal,
children: List.generate(10, (index) {
return ScrollContent(
id: '$index',
child: Container(
alignment: Alignment.center,
width: 200,
child: Text(
'$index',
style: TextStyle(color: Colors.white, fontSize: 50),
),
color: _colorList[index % _colorList.length],
),
);
}),
),
),
],
);
}
}
Flutterラボ
hatchoutschool
Flutter Daily
Flutterに関する記事を日々更新しています (222本)
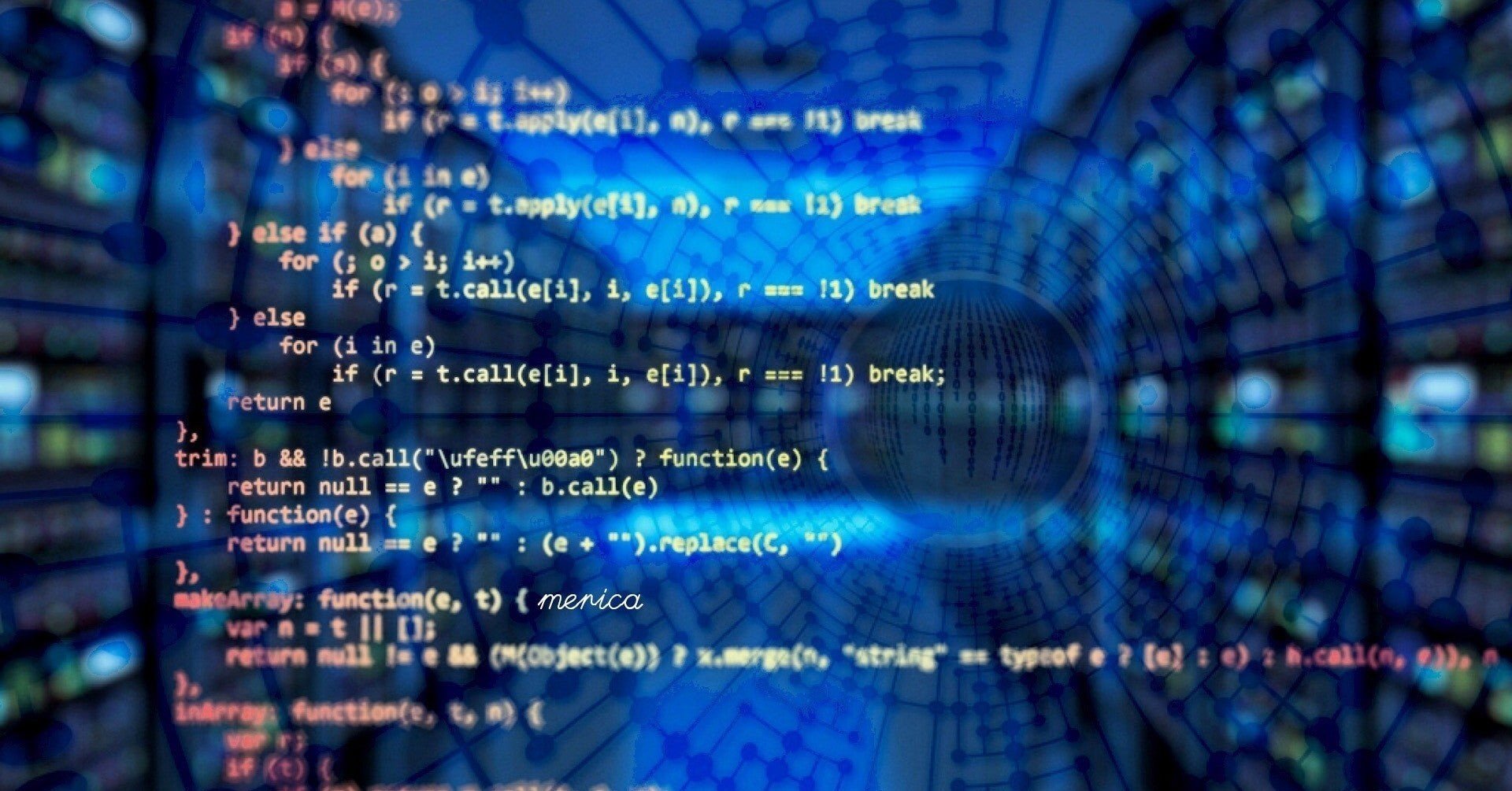
【Dart】Stringからint, double, DateTimeに変換する
2020.09.14
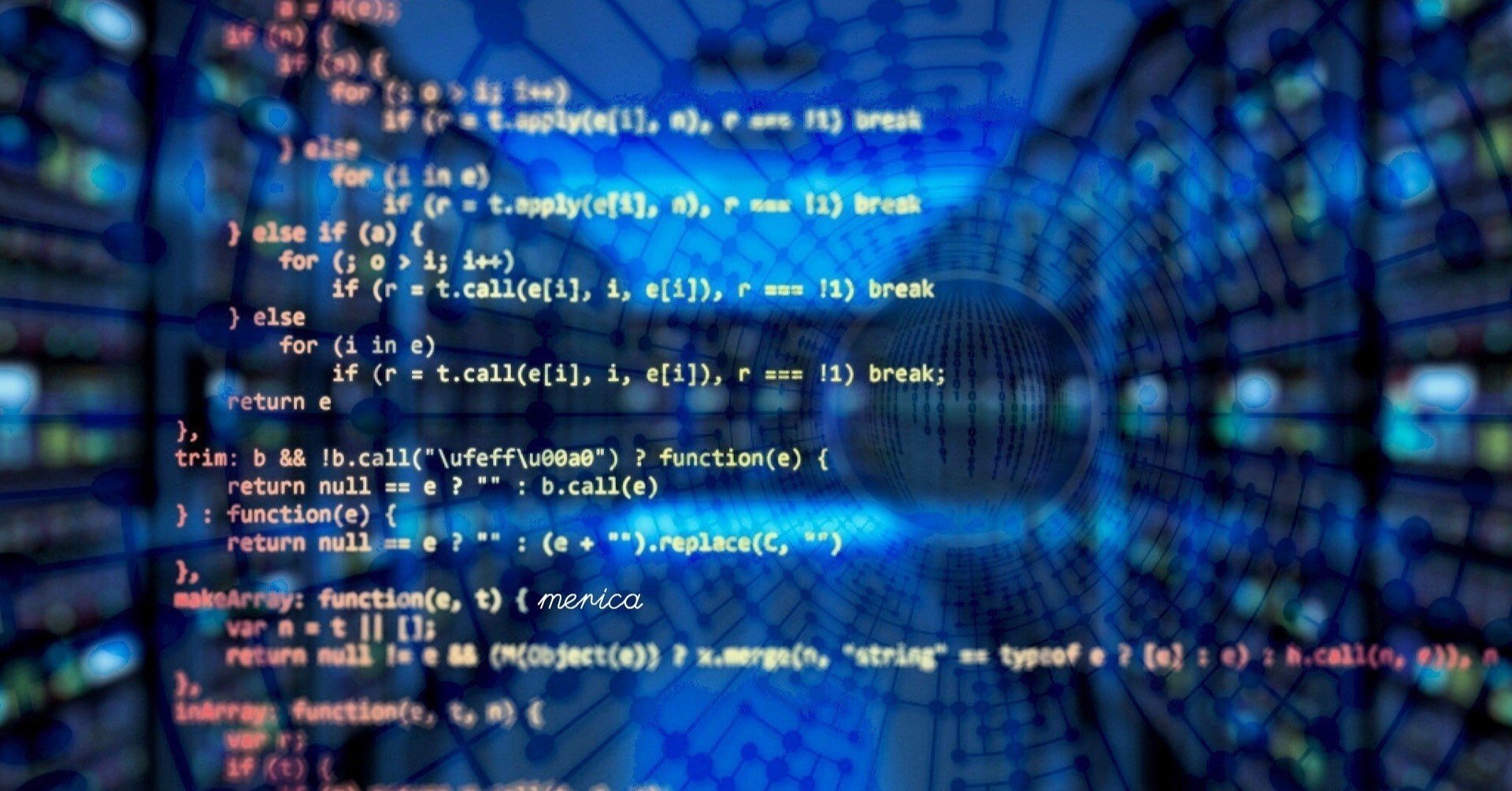
【Dart】【Flutter】List型(リスト)の使い方とよく使うメソッドまとめ
2020.09.18
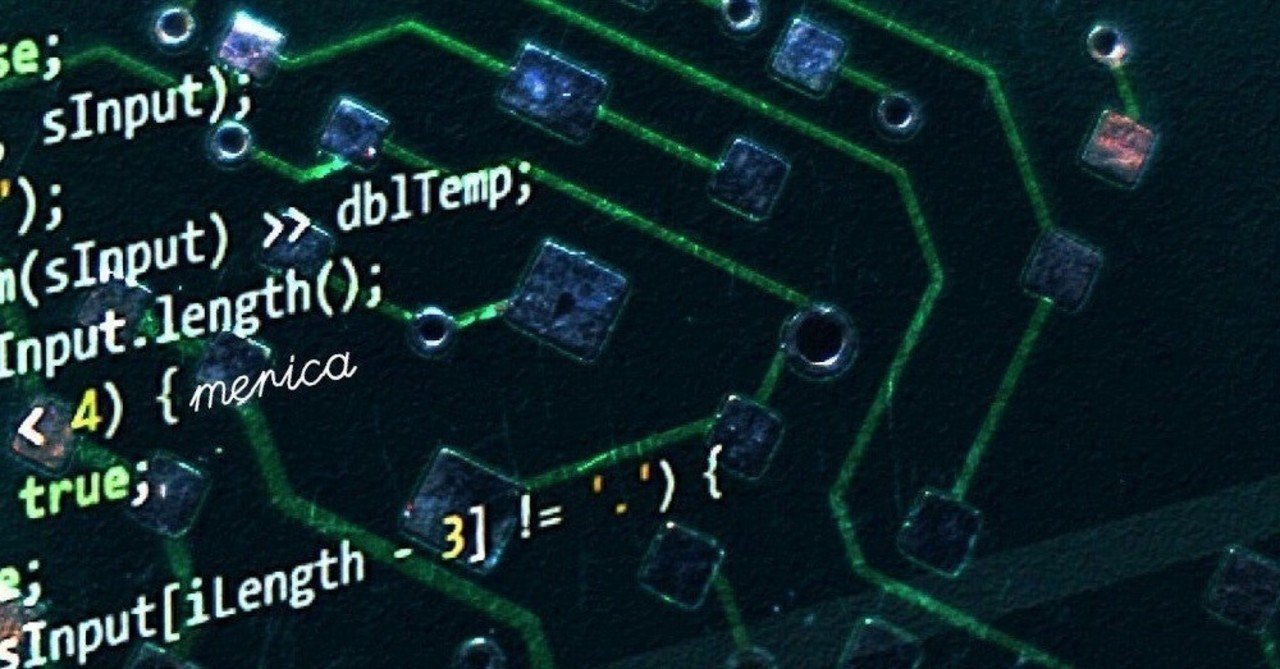
【Dart】【Flutter】DateTime型についてのまとめ
2020.10.01
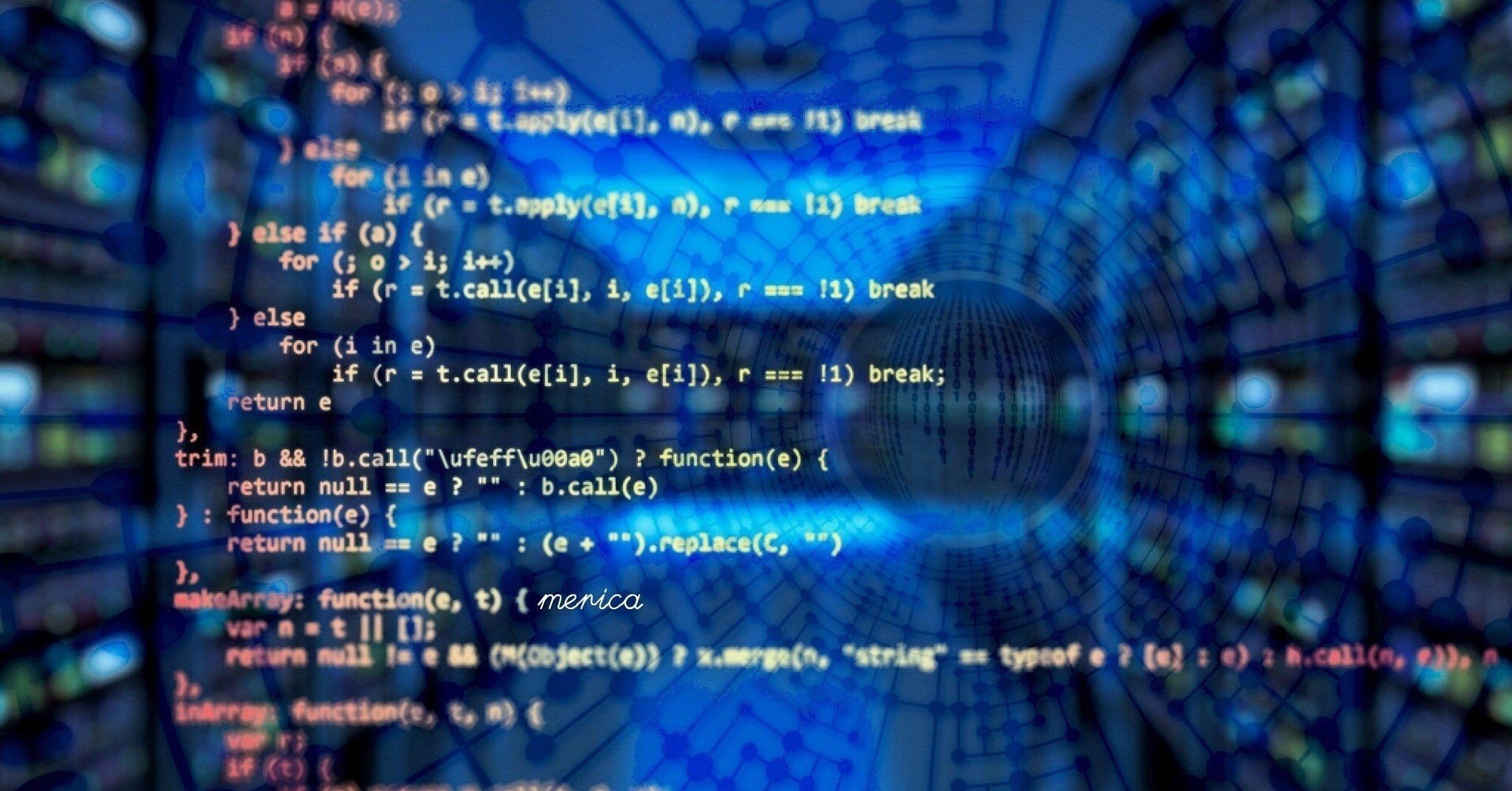
【Dart】Map型の使い方とよく使うメソッドまとめ
2020.09.13