Slackコミュニティに
無料で参加する
Flutterラボの
プレミアム会員になる
【Flutter】大学入学共通テスト(旧センター試験)のマークシートを作成してみた
2022.01.09
受験シーズンということで、マークシートをFlutterで作成してみました。
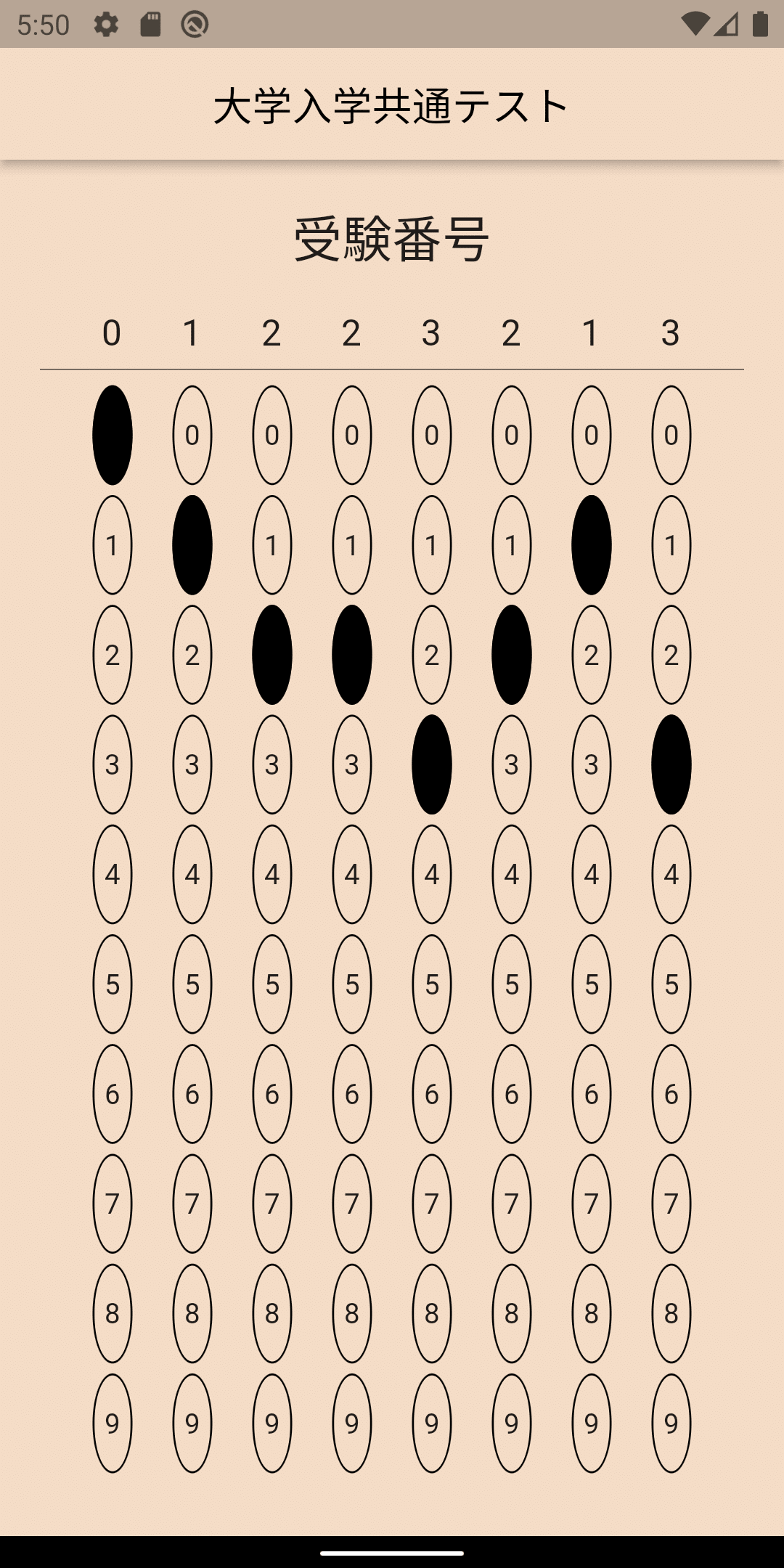
全体コード
import 'package:flutter/material.dart';
void main() async {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutterラボ',
theme: ThemeData(
primarySwatch: getMaterialColor(0XFFF4DCC6),
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: const CenterExamination(),
);
}
MaterialColor getMaterialColor(int colorCode) {
Map<int, Color> _map = {};
for(int i = 50; i < 1000; i += 50) {
_map[i] = Color(colorCode);
}
return MaterialColor(
colorCode,
_map
);
}
}
class CenterExamination extends StatefulWidget {
const CenterExamination({Key? key}) : super(key: key);
@override
_CenterExaminationState createState() => _CenterExaminationState();
}
class _CenterExaminationState extends State<CenterExamination> {
final List<int?> _selectedNumber = List.filled(8, null);
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: const Color(0XFFF4DCC6),
appBar: AppBar(
centerTitle: true,
title: const Text('大学入学共通テスト'),
),
body: Padding(
padding: const EdgeInsets.all(20.0),
child: Column(
children: [
const Text('受験番号', textAlign: TextAlign.center, style: TextStyle(fontSize: 25),),
const SizedBox(height: 20,),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: _selectedNumber.map((e) {
return Container(
alignment: Alignment.center,
width: 40,
child: Text((e ?? '').toString(), style: const TextStyle(fontSize: 18),)
);
}).toList(),
),
const Divider(color: Colors.black,),
...List.generate(10, (index) => index).map((x) {
return Padding(
padding: const EdgeInsets.only(bottom: 5),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: List.generate(8, (index) => index).map((y) {
return mark(x, y);
}).toList(),
),
);
}).toList()
],
),
)
);
}
/// x = 数字, y = 左から何番目か
Widget mark(int x, int y) {
return GestureDetector(
child: Container(
alignment: Alignment.center,
width: 40,
child: Container(
margin: const EdgeInsets.symmetric(horizontal: 5),
width: 20,
height: 50,
child: Text('$x'),
alignment: Alignment.center,
decoration: BoxDecoration(
color: _selectedNumber[y] == x ? Colors.black : Color(0XFFF4DCC6),
border: Border.all(width: 1),
borderRadius: const BorderRadius.all(Radius.elliptical(20, 50))
),
),
),
onTap: () {
setState(() {
_selectedNumber[y] = x;
});
},
);
}
}
Flutterラボ
hatchoutschool
Flutter Daily
Flutterに関する記事を日々更新しています (223本)
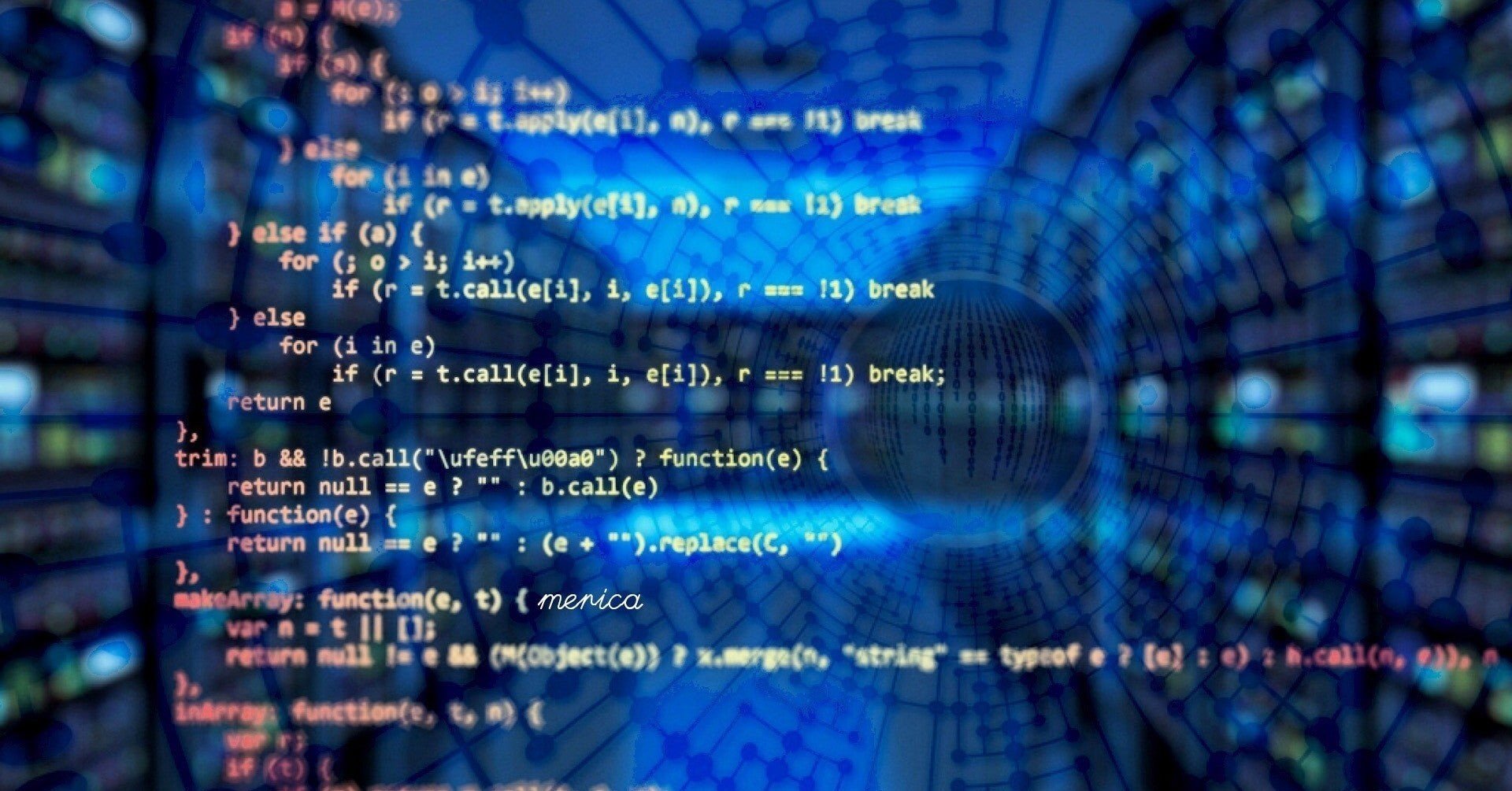
【Dart】Stringからint, double, DateTimeに変換する
2020.09.14
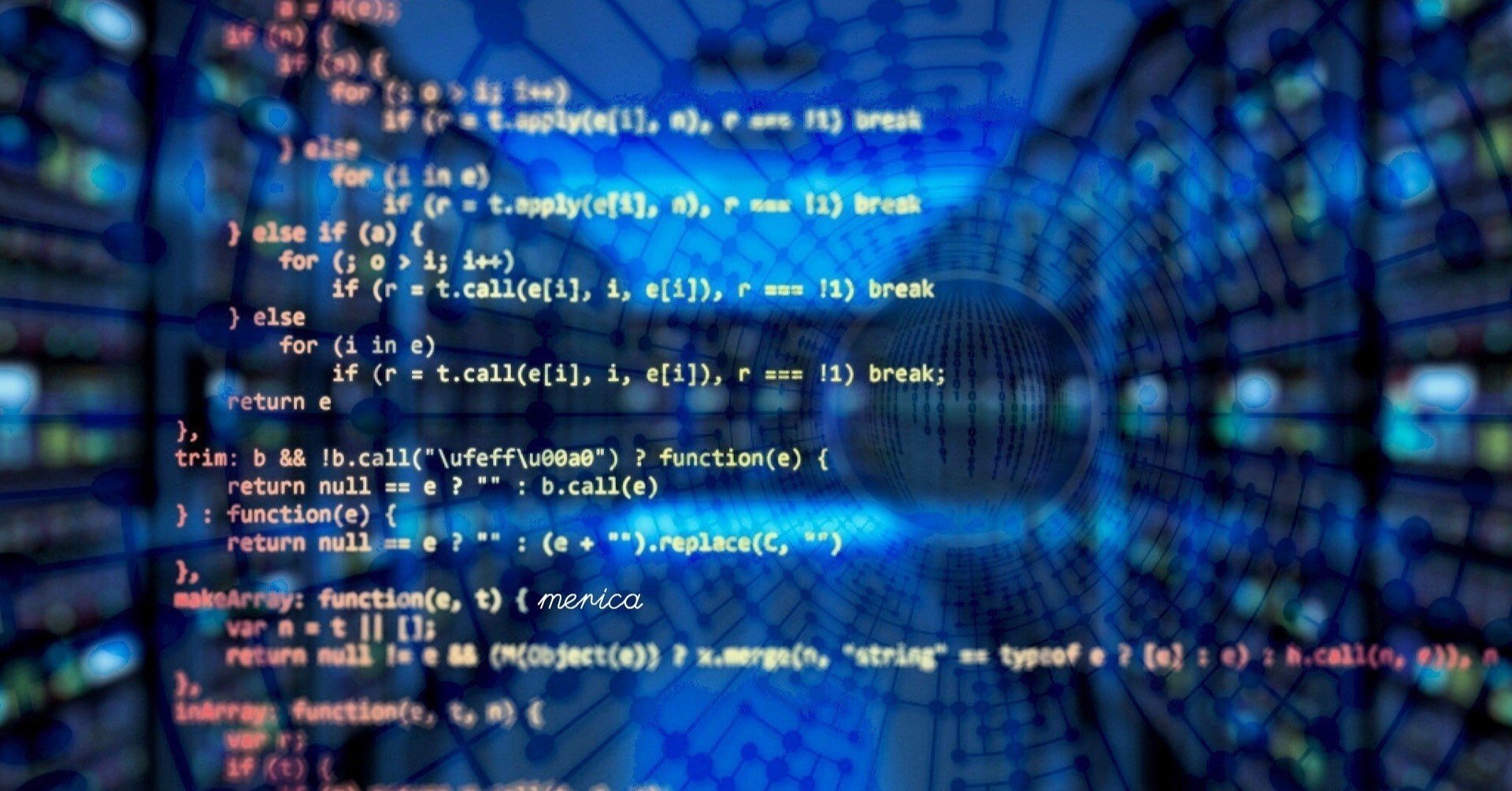
【Dart】【Flutter】List型(リスト)の使い方とよく使うメソッドまとめ
2020.09.18
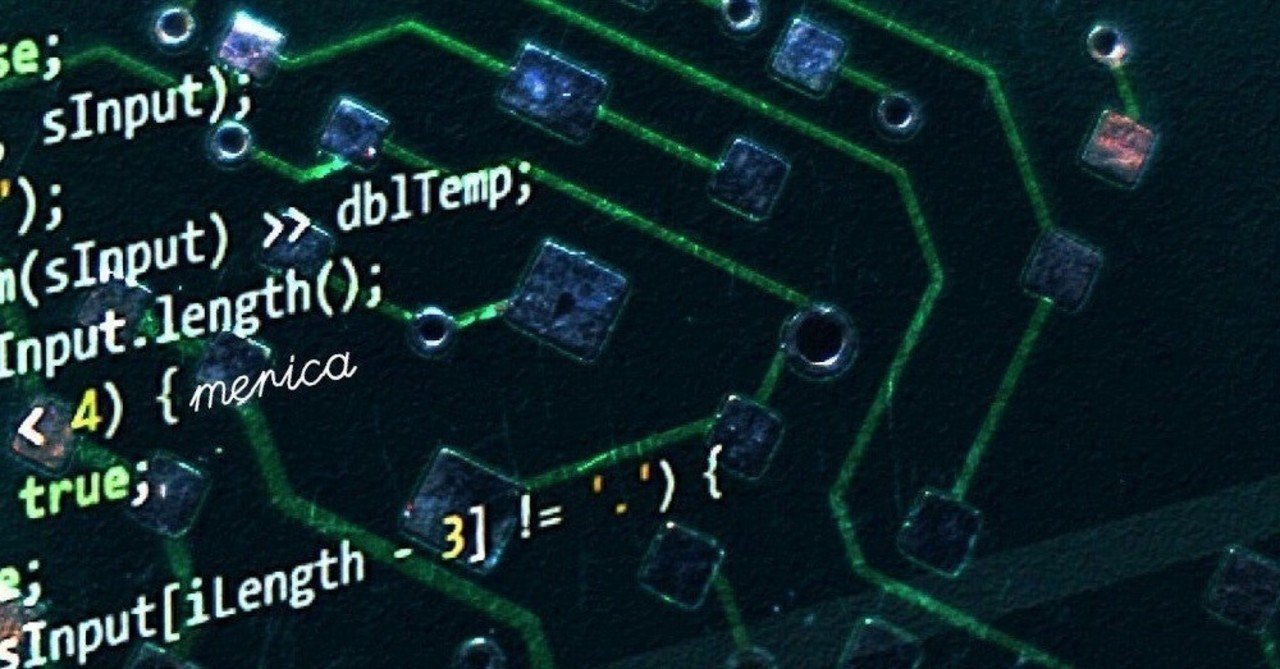
【Dart】【Flutter】DateTime型についてのまとめ
2020.10.01
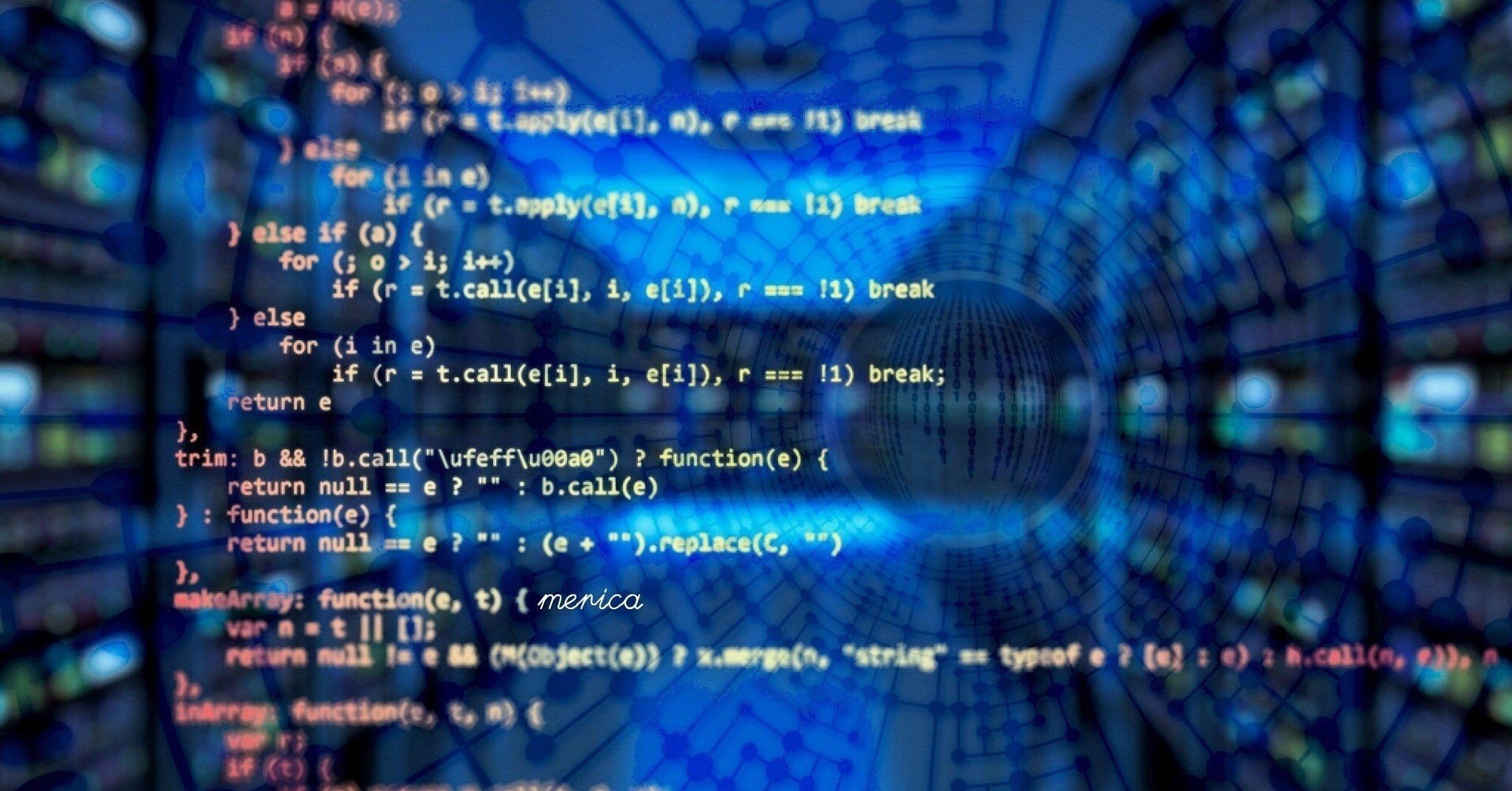
【Dart】Map型の使い方とよく使うメソッドまとめ
2020.09.13