広告
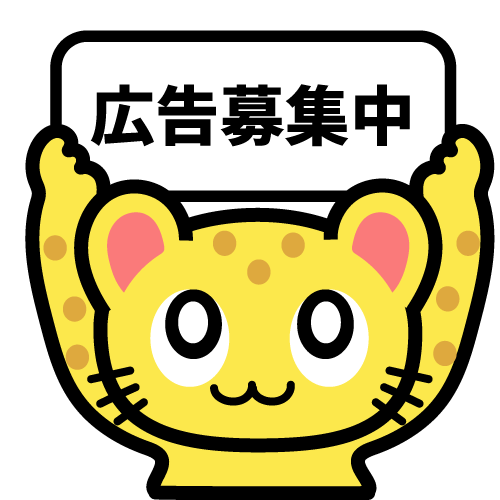
Flutterラボの
プレミアム会員になる
【Flutter】【Dart】端末サイズからWidgetのサイズを指定する
2020.08.19
端末のサイズデータから画面に表示したいWidgetのサイズを指定したいことってありませんか?しかし、それぞれの端末ごとに分岐させて〇〇pxのように指定してしまうと、とてつもない作業量になります。こちらの記事ではMediaQueryを使用して、端末のサイズを取得しそれぞれのWidgetのサイズを指定していきたいと思います。
サイズを決め打ちで入力した時の問題点
body: Padding(
padding: const EdgeInsets.all(8.0),
child: Center(
child: Column(
children: [
Container(
height: 100,
color: Colors.blue,
),
Container(
height: 200,
color: Colors.green,
),
Container(
height: 300,
color: Colors.red,
),
],
),
),
),
Pixel2の場合(MediaQuery使用前)
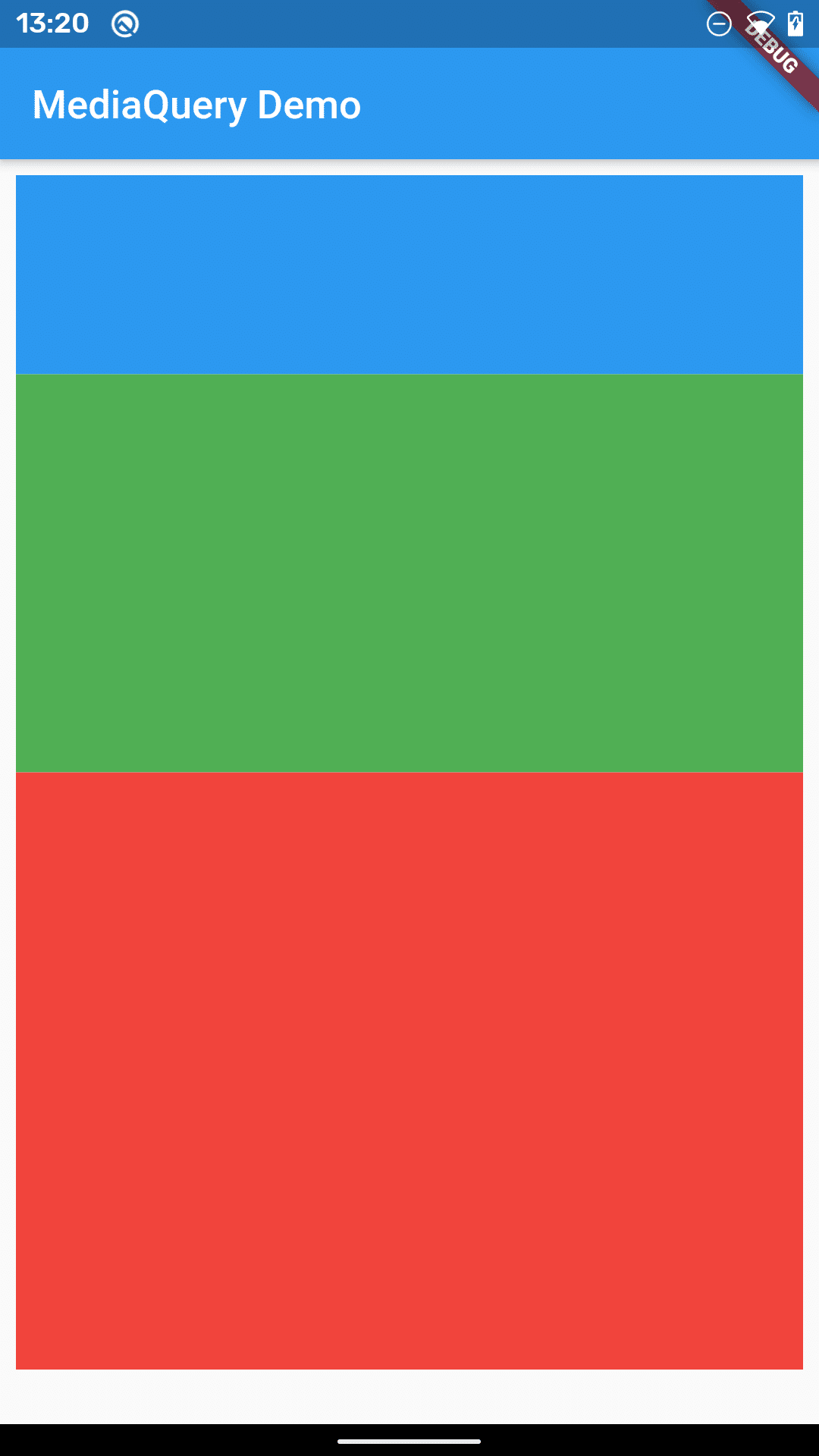
iPhone6sの場合(MediaQuery使用前)
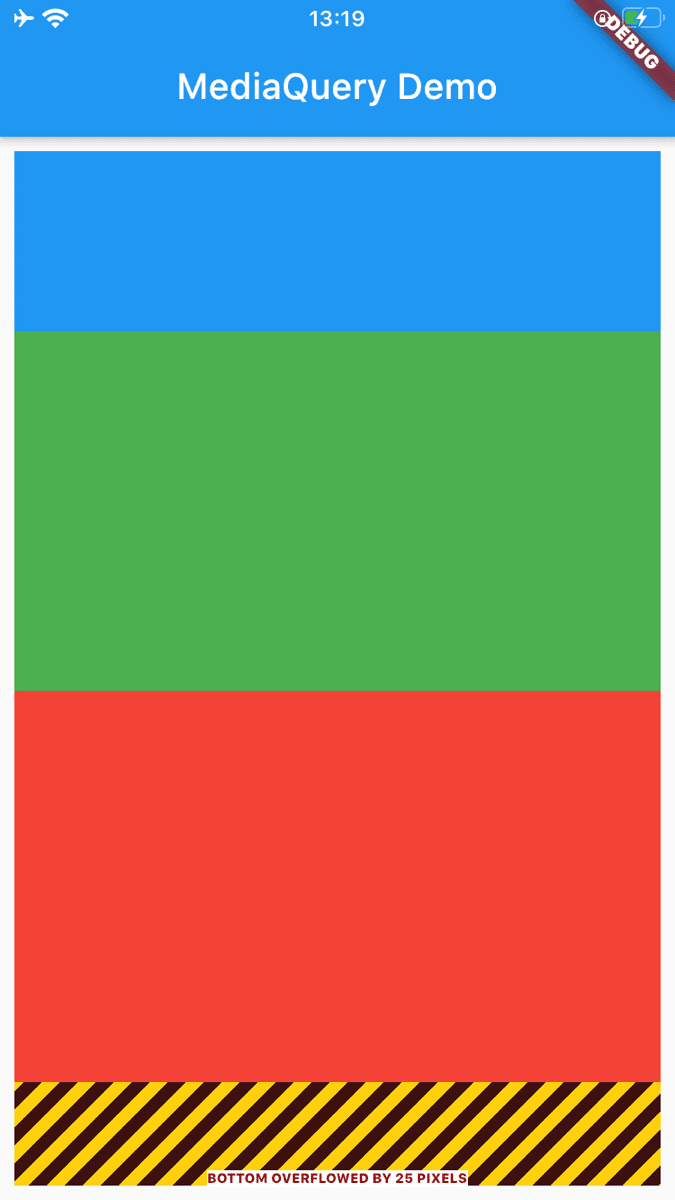
上のように画面の大きいデバイスだと問題ないのですが、iPhone6sのように画面の小さいデバイスだとエラーが出てしまいます。
MediaQueryをBuild内で使用して変数に代入しておく
Widget build(BuildContext context) {
final double deviceHeight = MediaQuery.of(context).size.height;
return Scaffold(
...
);
}
使用したい場所で画面との割合と計算して変数を呼び出す。
Padding(
padding: const EdgeInsets.all(8.0),
child: Center(
child: Column(
children: [
Container(
height: deviceHeight * 0.1,
color: Colors.blue,
),
Container(
height: deviceHeight * 0.3,
color: Colors.green,
),
Container(
height: deviceHeight * 0.4,
color: Colors.red,
),
],
),
),
),
Pixel2(MediaQuery使用後)
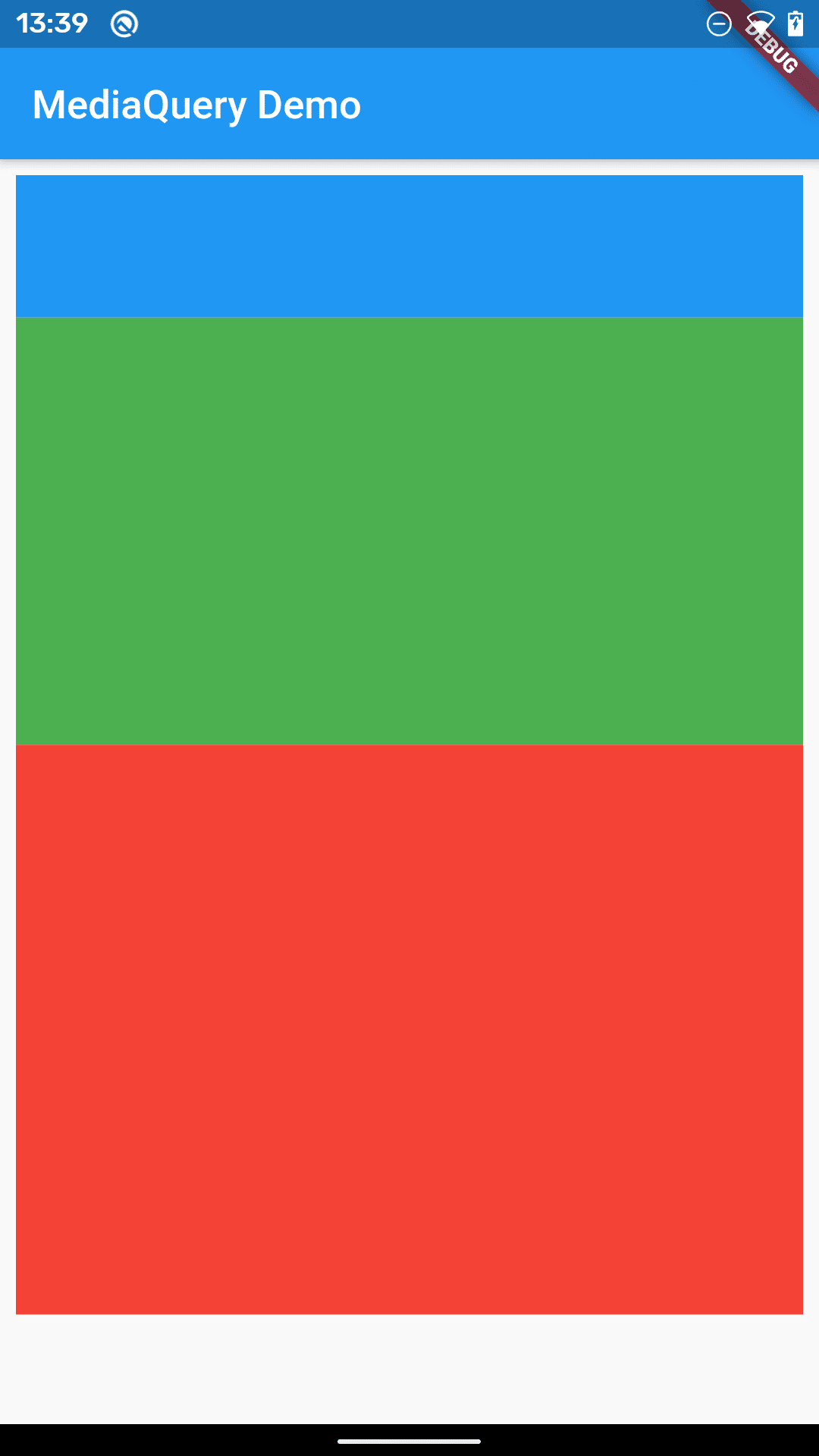
iPhone6sでは(MediaQuery使用後)
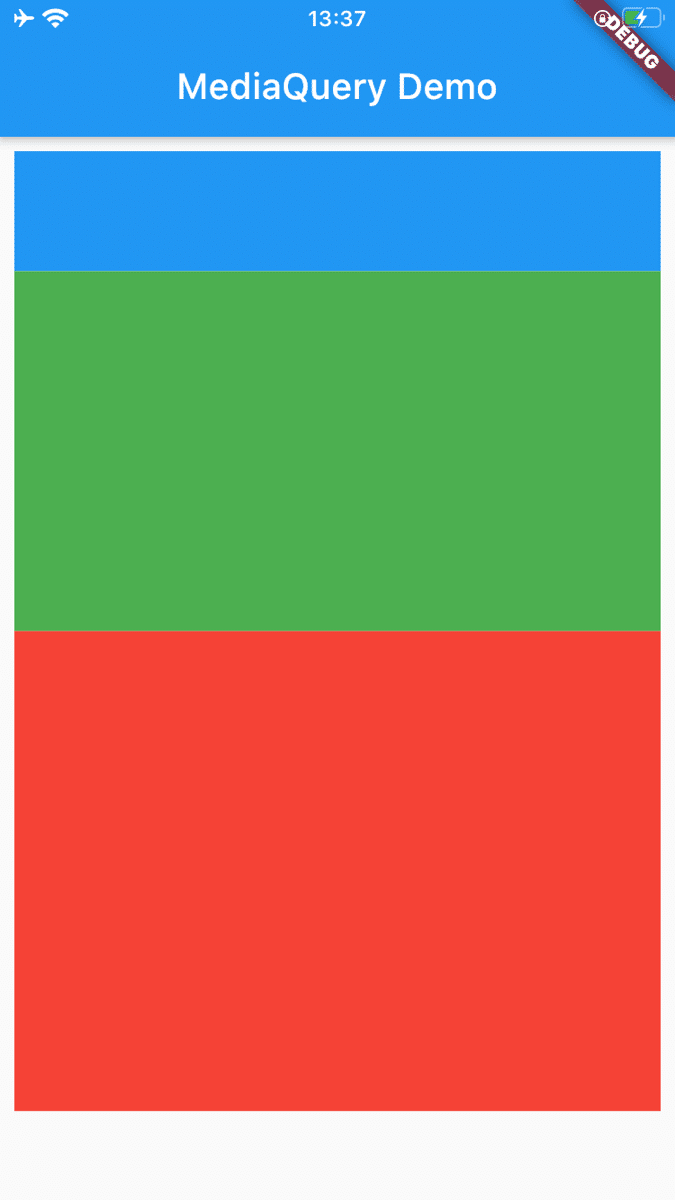
全体コード
class HomePage extends StatefulWidget {
HomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
final double deviceHeight = MediaQuery.of(context).size.height;
return Scaffold(
resizeToAvoidBottomPadding: false,
appBar: AppBar(
title: Text(widget.title),
),
body: Padding(
padding: const EdgeInsets.all(8.0),
child: Center(
child: Column(
children: [
Container(
height: deviceHeight * 0.1,
color: Colors.blue,
),
Container(
height: deviceHeight * 0.3,
color: Colors.green,
),
Container(
height: deviceHeight * 0.4,
color: Colors.red,
),
],
),
),
),
);
}
}
Flutterラボ
hatchoutschool
Flutter Daily
Flutterに関する記事を日々更新しています (222本)
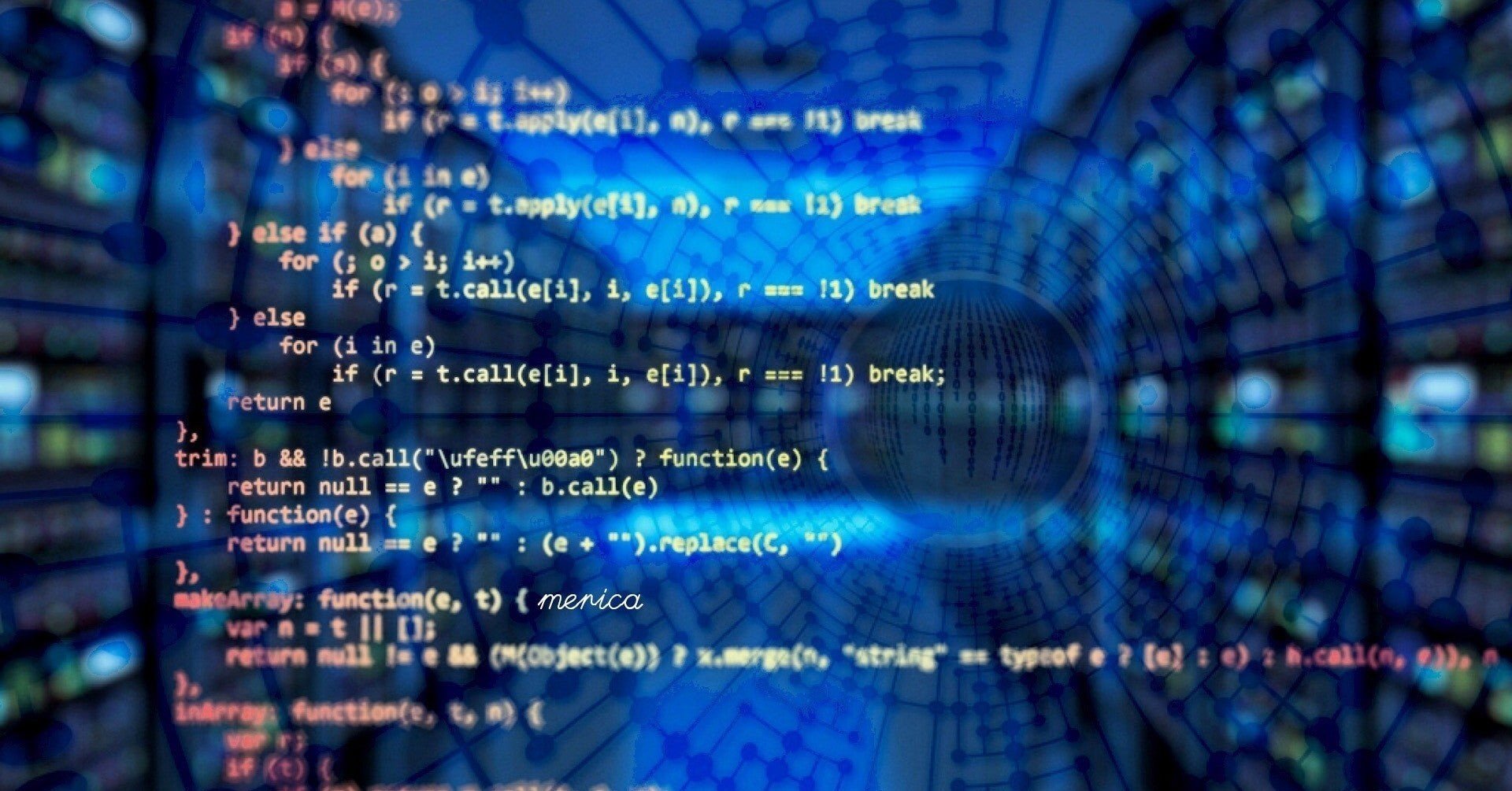
【Dart】Stringからint, double, DateTimeに変換する
2020.09.14
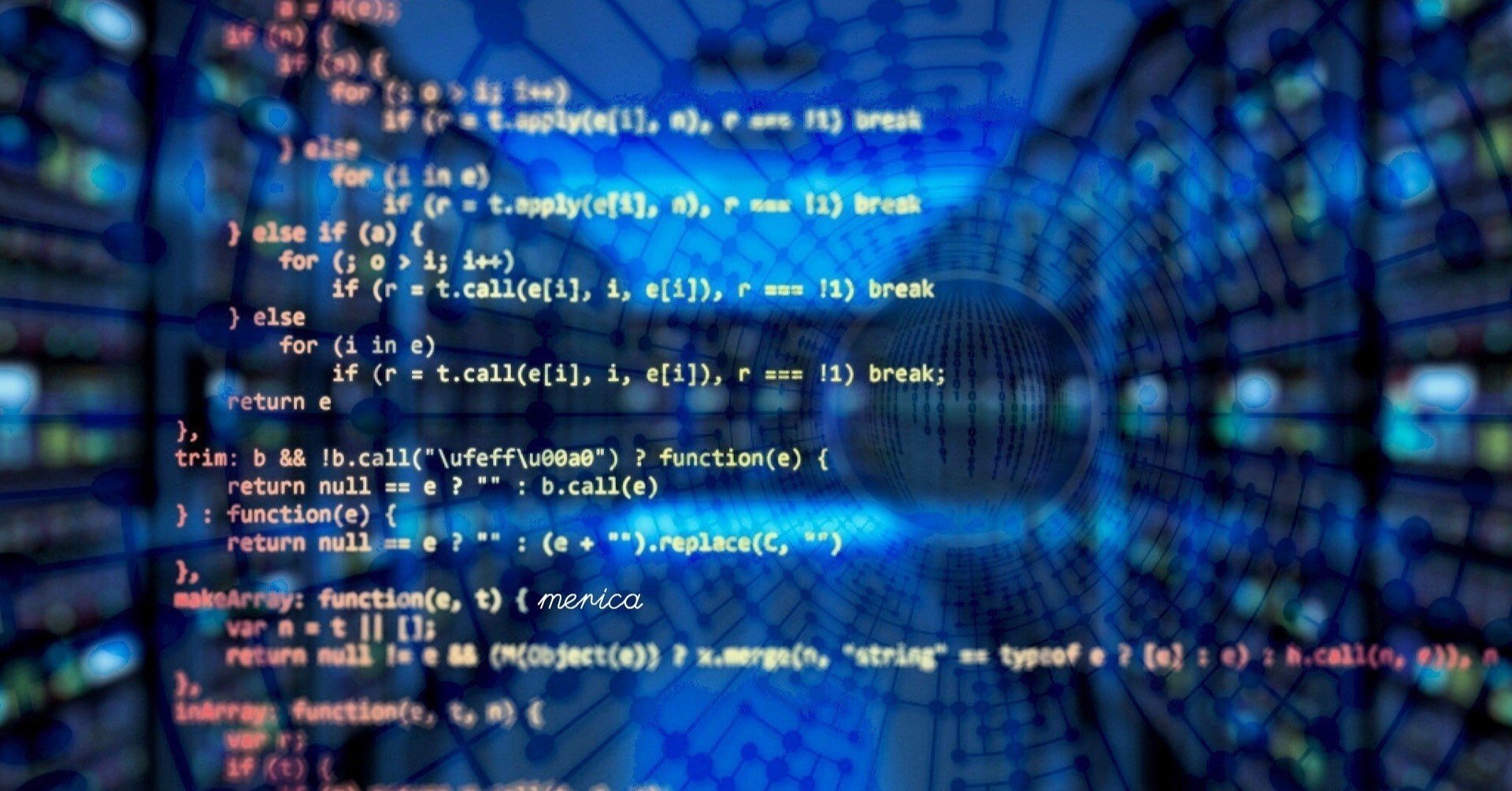
【Dart】【Flutter】List型(リスト)の使い方とよく使うメソッドまとめ
2020.09.18
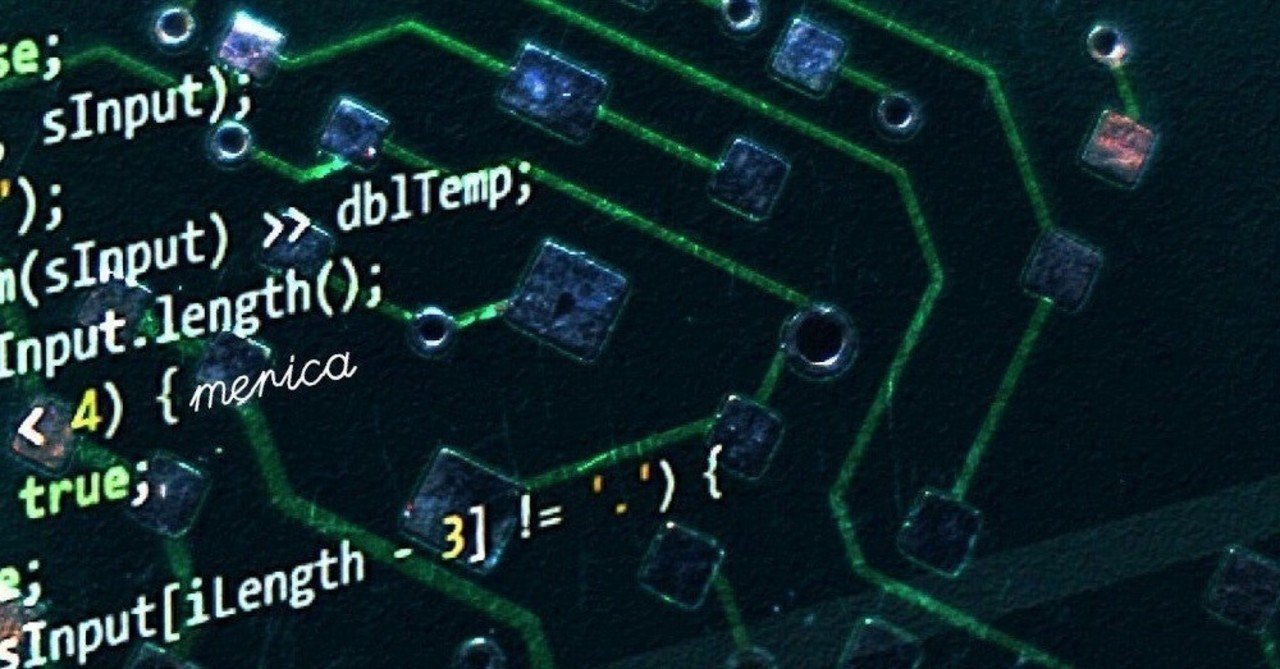
【Dart】【Flutter】DateTime型についてのまとめ
2020.10.01
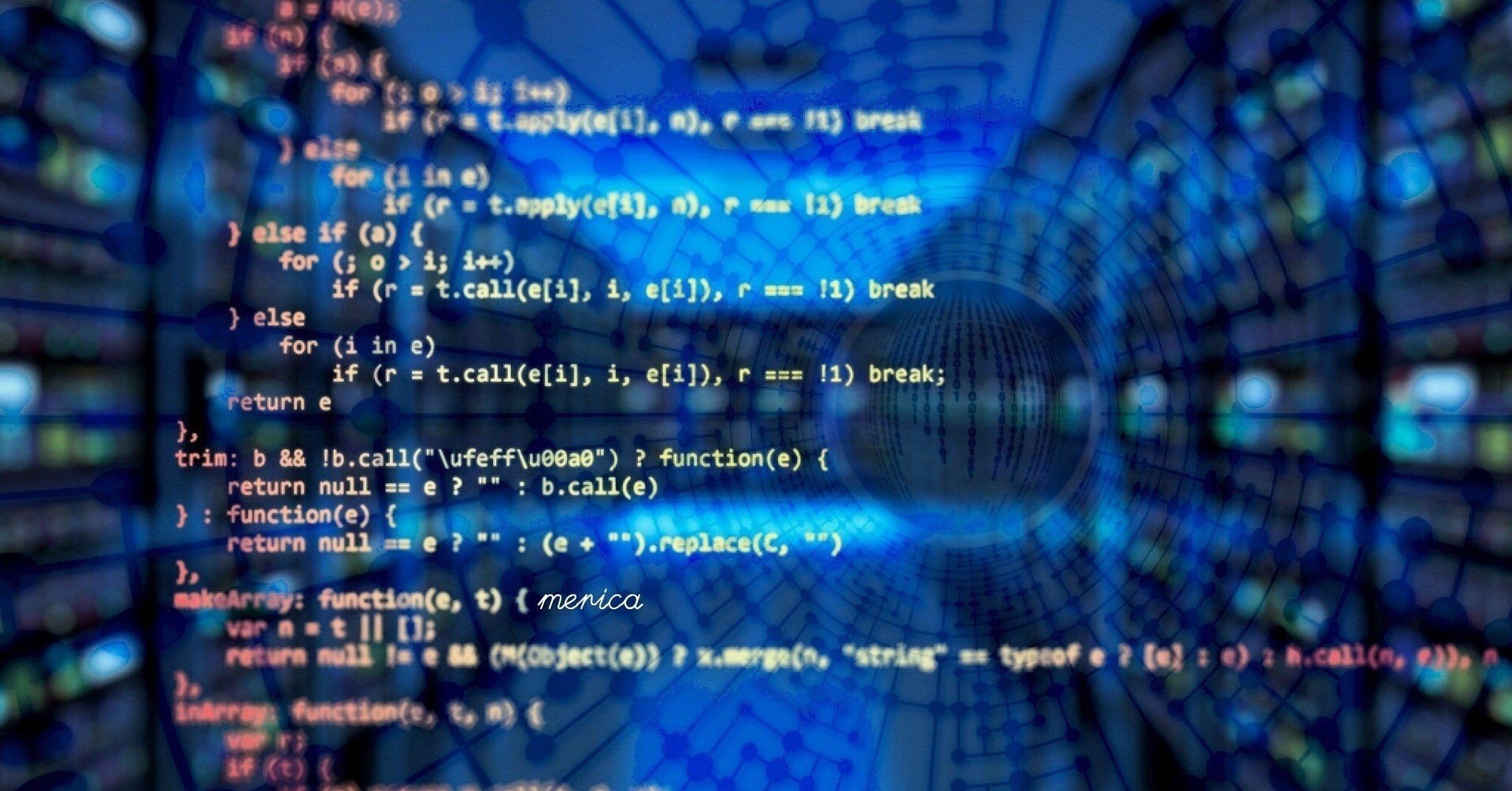
【Dart】Map型の使い方とよく使うメソッドまとめ
2020.09.13